First, you have an app that shows an activity that consists of a single screen with a text field and a "Send" button after you complete the previous lesson(Android Studio-02). In this lesson shows you how to start a new activity to display a message when taps the "Send" button.
【MainActivity】 As the following code:
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.EditText;
public class MainActivity extends AppCompatActivity { public static final String EXTRA_MESSAGE = "com.example.myfirstapp.MESSAGE"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void sendMessage(View view) { Intent intent = new Intent(this, DisplayMessageActivity.class); EditText editText = (EditText) findViewById(R.id.editTextTextPersonName); String message = editText.getText().toString(); intent.putExtra(EXTRA_MESSAGE, message); startActivity(intent); } }
|
【activity_main.xml】
Click Attributes window → onClick →Select sendMessage from its drop-down list
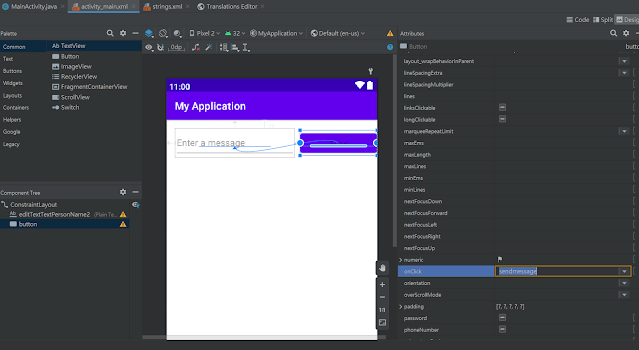
【DisplayMessageActivity】
To create the second activity
Project window → Right-Click the app folder
→ Select New → Activity
→ Select Empty Activity → DisplayMessageActivity3
As the following code:
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.widget.TextView;
public class DisplayMessageActivity extends AppCompatActivity {
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_display_message);
// Get the Intent that started this activity and extract the string Intent intent = getIntent(); String message = intent.getStringExtra(MainActivity.EXTRA_MESSAGE);
// Capture the layout's TextView and set the string as its text TextView textView = findViewById(R.id.textView); textView.setText(message);
} }
|
【AndroidManifest.xml】
As the following code:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.myapplication">
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.MyApplication"> <activity android:name=".DisplayMessageActivity" android:parentActivityName=".MainActivity"/> <!-- The meta-data tag is required if you support API level 15 and lower --> <meta-data android:name="android.support.PARENT_ACTIVITY" android:value=".MainActivity" /> <activity android:name=".MainActivity" android:exported="true"> <intent-filter> <action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application>
</manifest>
|
Run the app on an emulator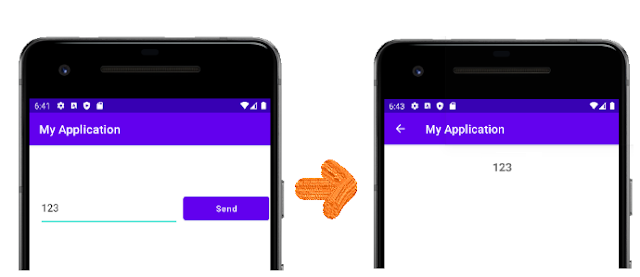